简介
说明
本文用示例介绍Spring如何将组件注入到静态工具类。
需求
在Controller层想使用一个静态工具,这个静态工具要使用其他组件。
错误方法:在静态字段上注入
Controller
package com.example.controller; import com.example.component.WelcomeUtil; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @RestController public class HelloController { @GetMapping("/test") public String test() { WelcomeUtil.welcome(); return "test success"; } }
工具类
package com.example.component; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Component; @Component public class WelcomeUtil { @Autowired private static Hello hello; public static void welcome() { hello.sayHello(); } }
引入的组件
package com.example.component; import org.springframework.stereotype.Component; @Component public class Hello { public void sayHello() { System.out.println("Hello"); } }
测试
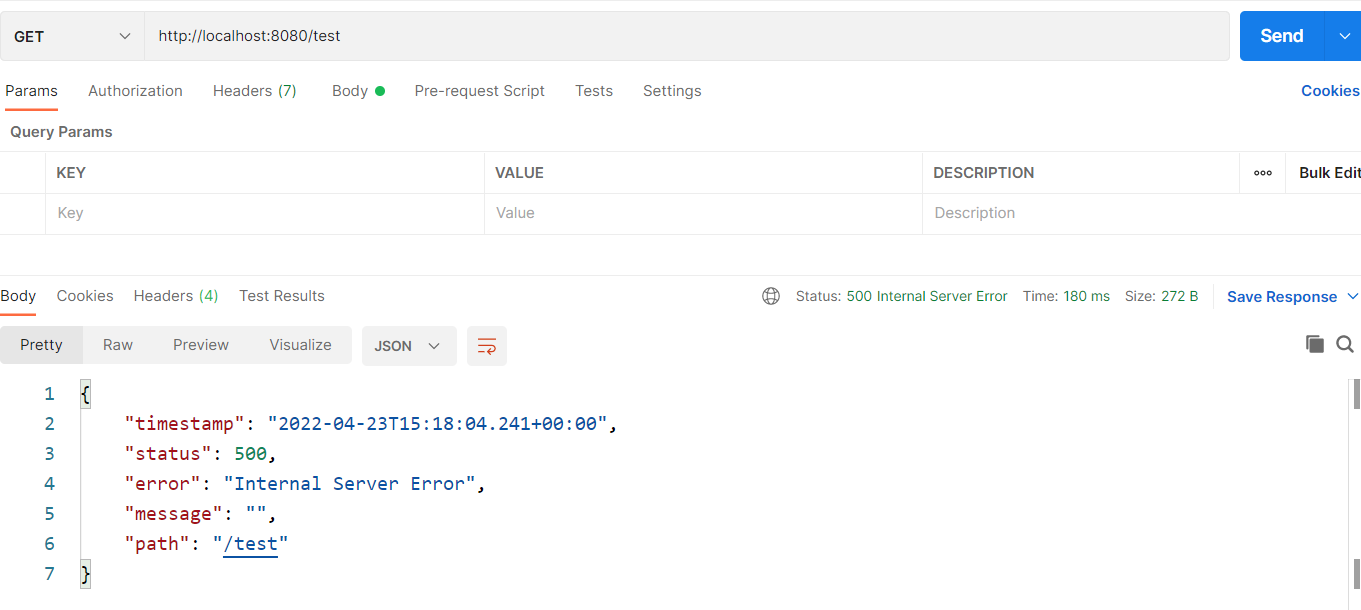
后端结果

方案1:构造器注入
其余代码与上边相同
工具类
package com.example.component; import org.springframework.stereotype.Component; @Component public class WelcomeUtil { private static Hello hello; public WelcomeUtil(Hello hello) { WelcomeUtil.hello = hello; } public static void welcome() { hello.sayHello(); } }
测试
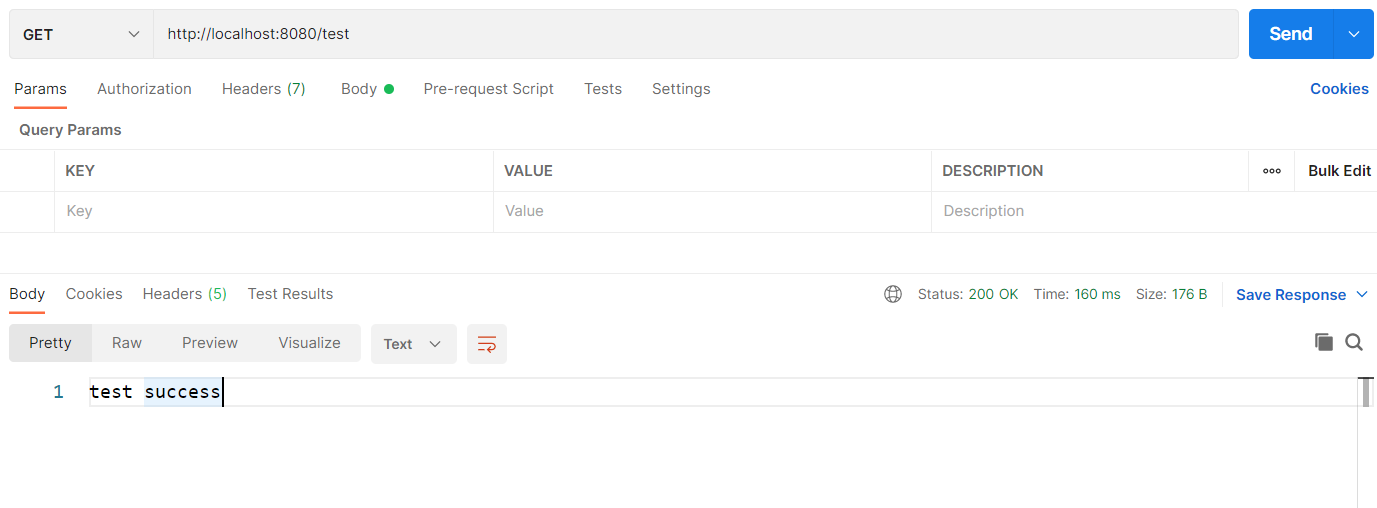
后端结果

方案2:setter注入
其余代码与上边相同
工具类
package com.example.component; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Component; @Component public class WelcomeUtil { private static Hello hello; public static void welcome() { hello.sayHello(); } @Autowired public void setHello(Hello hello) { WelcomeUtil.hello = hello; } }
测试
结果
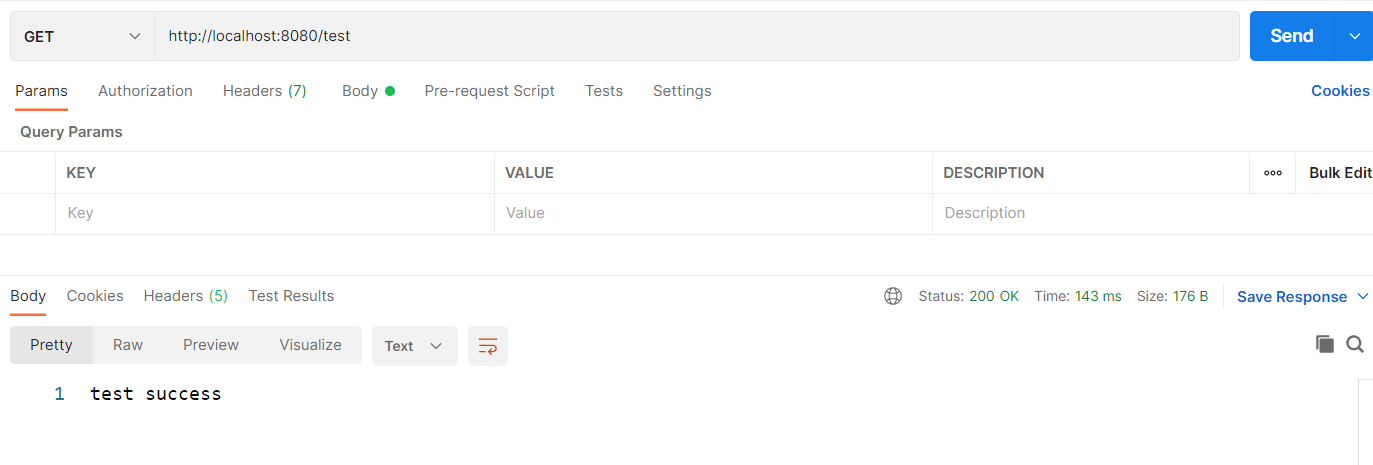
后端结果

方案3:ApplicationContext工具类
ApplicationContext工具类
package com.example.util; import org.springframework.beans.BeansException; import org.springframework.context.ApplicationContext; import org.springframework.context.ApplicationContextAware; import org.springframework.stereotype.Component; @Component public class ApplicationContextHolder implements ApplicationContextAware { private static ApplicationContext context; @Override public void setApplicationContext(ApplicationContext context) throws BeansException { ApplicationContextHolder.context = context; } public static ApplicationContext getContext() { return context; } }
工具类
package com.example.component; import com.example.util.ApplicationContextHolder; import org.springframework.stereotype.Component; @Component public class WelcomeUtil { public static void welcome() { ApplicationContextHolder.getContext() .getBean(Hello.class).sayHello(); } }
测试
结果
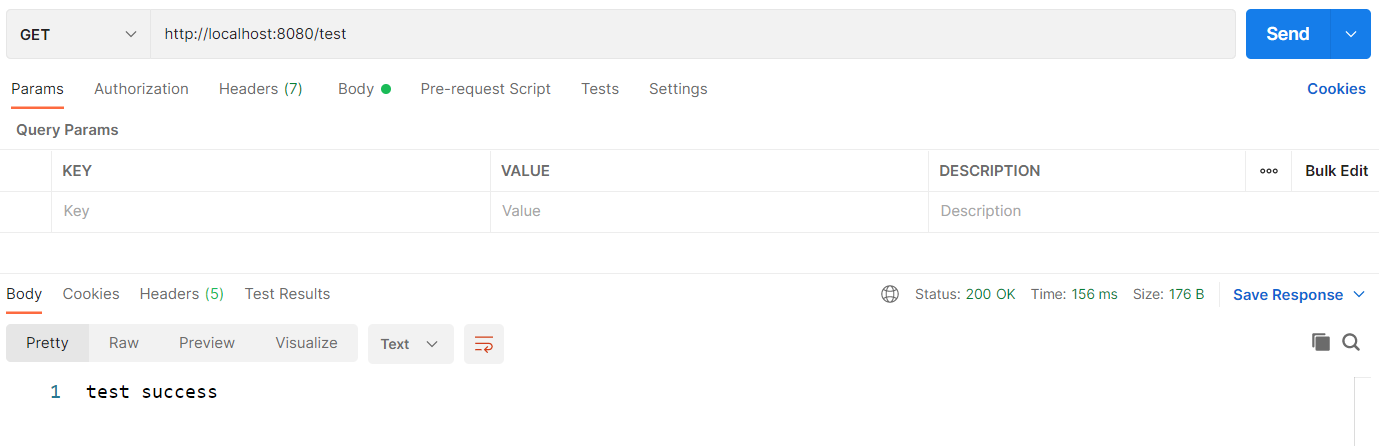
后端结果

请先
!